As an automation QA, I usually set up test frameworks from scratch using POM design pattern with OOP structure. That's a good approach where you can control everything in your framework. However, if the project is limited in terms of time or your team members are not really good with coding, then you may need a "portable" framework where tests can be written and run quickly without much too much base coding.
Karate is built on top of Cucumber so you'll mainly write your test cases in Gherkin language. Besides, you can also write custom scripts using other languages such as Java and Javascript. Karate is a universal tool where it supports many different testing types, for examples: API test, UI test, even Performance test.
1. Set up pre-requisites
In order to work with Karate smoothly, you may need to set up these dependencies on your local machine first:
Libraries | |
---|---|
JDK |
- Downloads - Guidelines (Windows) - Guidelients (MacOS) |
Maven |
- Downloads - Guidelines (Windows) - Guidelines (MacOS) |
IDE | |
Visual Studio Code |
I prefer this one since it’s easier for running/debugging tests. Extensions: - Karate Runner - Cucumber (Gherkin) Full Support - Karate Formatter |
IntelliJ IDEA | IntelliJ IDEA is good but you need to pay for Karate plugin in order to debug. |
2. Set up a Karate test project from scratch
Below set up is done using Visual Studio Code, and steps should be similar for any other IDEs. Reference: https://github.com/karatelabs/karate#getting-started
1. Create a new folder, name it “integration_tests” for example.
2. Under “integration_tests”:
- Create a new directory as “/src/test/java”.
- Add a new “pom.xml” file at “/integration_tests".
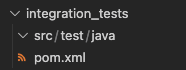
3. In pom.xml, put this content:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.fundingsocieties</groupId>
<artifactId>my-integration-tests</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>jar</packaging>
<properties>
<java.version>11</java.version>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.version>3.8.1</maven.compiler.version>
<maven.surefire.version>2.22.2</maven.surefire.version>
<karate.version>1.3.1</karate.version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencies>
<dependency>
<groupId>com.intuit.karate</groupId>
<artifactId>karate-junit5</artifactId>
<version>${karate.version}</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<testResources>
<testResource>
<directory>src/test/java</directory>
<excludes>
<exclude>**/*.java</exclude>
</excludes>
</testResource>
</testResources>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>${maven.compiler.version}</version>
<configuration>
<encoding>UTF-8</encoding>
<source>${java.version}</source>
<target>${java.version}</target>
<compilerArgument>-Werror</compilerArgument>
</configuration>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>${maven.surefire.version}</version>
<configuration>
<argLine>-Dfile.encoding=UTF-8</argLine>
</configuration>
</plugin>
</plugins>
</build>
</project>
4. Right click inside of pom.xml, select “Reload Projects” for dependencies to be downloaded to your local machine.
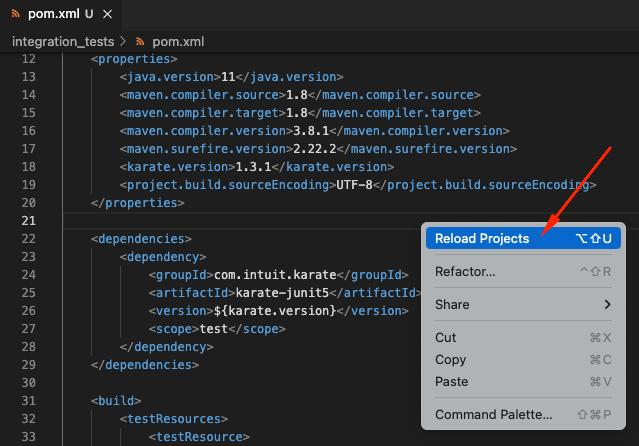
5. Add “karate-config.js” to “/src/test/java”. karate.env is a system property and can be set when executing command line. Reference: https://github.com/karatelabs/karate#karate-configjs.
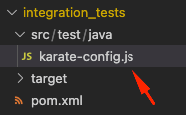
function fn() {
const env = karate.env;
let config = {
baseUrl: null
};
if (env === 'local') {
config.baseUrl = 'http://localhost:8181/v1';
} else if (env === 'uat') {
// TODO:
}
karate.configure('connectTimeout', 5000);
karate.configure('readTimeout', 5000);
return config;
}
6. Add “logback-test.xml” to “/src/test/java”. This holds the configuration for log information/format to the console.
<?xml version="1.0" encoding="UTF-8"?>
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<appender name="FILE" class="ch.qos.logback.core.FileAppender">
<file>target/karate.log</file>
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<logger name="com.intuit.karate" level="DEBUG" />
<root level="info">
<appender-ref ref="STDOUT" />
<appender-ref ref="FILE" />
</root>
</configuration>
7. Now, let’s add:
- “TestRunner.java” to “/src/test/java”.
- “test.feature” to “/src/test/java/features”.
8. Content of “TestRunner.java“:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
import com.intuit.karate.Results;
import com.intuit.karate.Runner;
public class TestRunner {
@Test
void runTest() {
Results results = Runner.path("classpath:features")
.tags("~@ignore")
.parallel(5);
assertEquals(0, results.getFailCount(), results.getErrorMessages());
}
}
9. Content of “test.feature“ should be very simple, just to prove that our code is able to be up and run.
Feature: Test
Scenario: Test
* karate.log('Hello World')
10. Now, let’s run our very first test:
- Click “Karate: Run” Codelens.
- Type “TestRunner -Dkarate.env=local” into popup input.
- Press Enter.
11. Congratulations, your very first test has been kicked off successfully. Now you’re ready to set off with Karate!!!